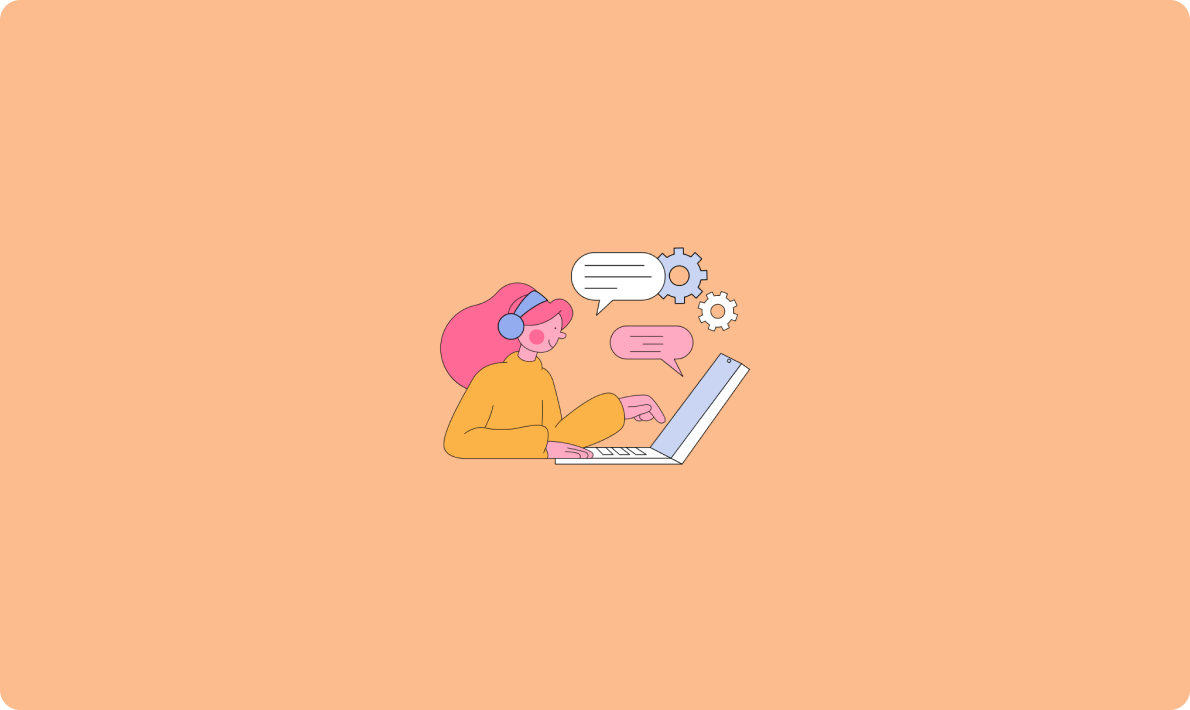
文心一言写代码:代码生成力的探索
文心一言(ERNIE Bot)是百度推出的一款强大生成式对话产品,具有广泛的应用代码生成能力。本文将详细介绍文心一言在实际应用中的代码能力及其在不同场景中的应用示例,包括如何调用API、实现代码生成,以及如何确保数据一致性等技术细节。同时,将针对开发者常见的问题进行解答,并提供相关图片链接和代码示例以支持理解。
文心一言不仅能够理解和生成自然语言文本,还可以根据用户需求生成高质量的代码解决方案。以下是其主要的代码能力:
在实际应用中,保证缓存和数据库的同步是一个经典挑战。下面我们以一个Java示例展示如何使用文心一言实现Redis与数据库的数据一致性。
首先,配置pom.xml
以引入Spring Boot和Redis依赖:
org.springframework.boot
spring-boot-starter-data-jpa
org.springframework.boot
spring-boot-starter-data-redis
接下来,创建实体类Inventory
用于存储库存信息:
@Entity
public class Inventory {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
private String productId;
private int quantity;
// getters and setters
}
创建InventoryRepository
接口进行数据库操作:
public interface InventoryRepository extends JpaRepository {
}
使用InventoryService
实现与Redis交互:
@Service
public class InventoryService {
@Autowired
private StringRedisTemplate redisTemplate;
@Autowired
private InventoryRepository inventoryRepository;
@Transactional
public void addToInventory(String productId, int quantity) {
redisTemplate.opsForValue().increment(productId, quantity);
Inventory inventory = inventoryRepository.findByProductId(productId);
if (inventory != null) {
inventory.setQuantity(inventory.getQuantity() + quantity);
inventoryRepository.saveAndFlush(inventory);
} else {
Inventory newInventory = new Inventory();
newInventory.setProductId(productId);
newInventory.setQuantity(quantity);
inventoryRepository.saveAndFlush(newInventory);
}
}
}
在上述实现中,使用@Transactional
注解确保Redis和数据库的操作在同一个事务中执行。这样可以在数据库事务失败时回滚操作,从而保持数据一致性。
调用文心一言API需要做好前期准备,包括注册百度开发者账号、获取API Key和Secret Key。以下是详细步骤:
以下是Python调用文心一言API的示例代码:
import requests
import json
api_key = 'your_api_key'
secret_key = 'your_secret_key'
url = 'https://wenxin.baidu.com/api/v1/text_generation'
payload = {
'api_key': api_key,
'secret_key': secret_key,
'prompt': '请写一篇关于人工智能发展的文章'
}
response = requests.post(url, data=payload)
if response.status_code == 200:
result = response.json()
if result['status'] == 0:
print('生成的文本内容:')
print(result['data']['generated_text'])
else:
print('请求失败,错误信息:', result['message'])
else:
print('请求失败,状态码:', response.status_code)
问:文心一言可以生成哪些类型的代码?
问:如何确保生成代码的正确性?
问:API调用频率是否有限制?
问:如何处理API调用失败的情况?
问:如何获取API更新的最新信息?
以上内容详细介绍了文心一言的应用代码功能与实际操作指南,希望对开发者在使用过程中有所帮助。