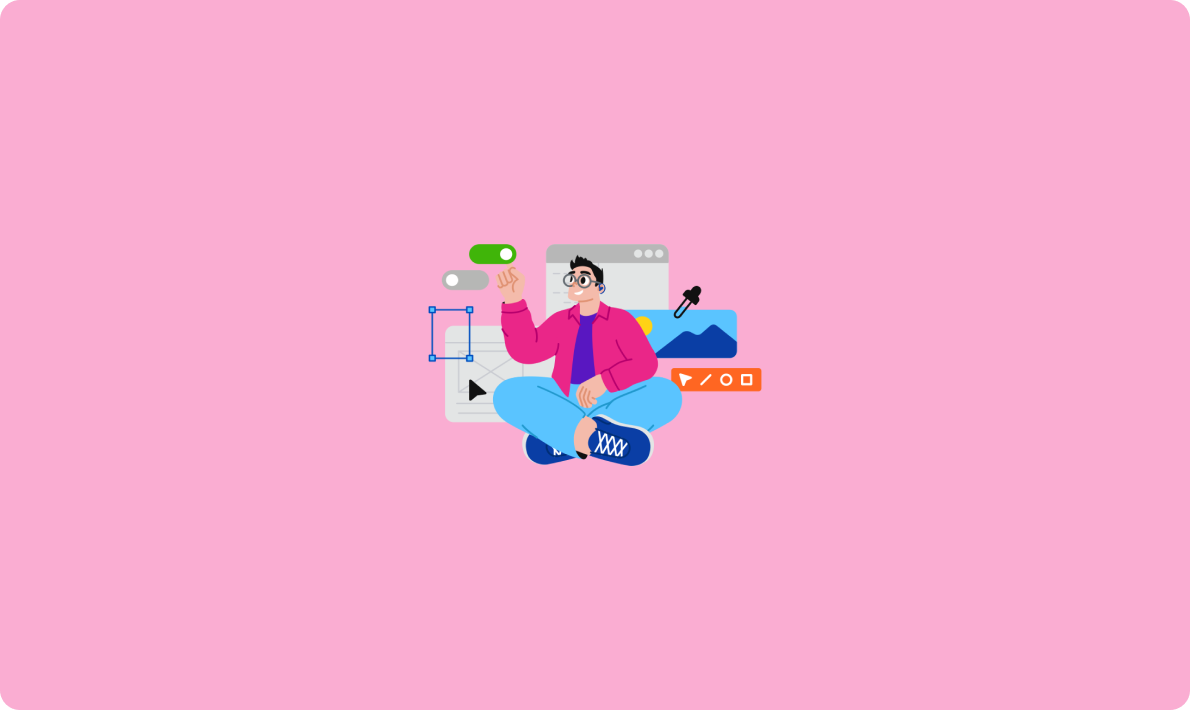
如何用AI进行情感分析
TensorFlow是一个强大的深度学习框架,本文将详细介绍如何使用TensorFlow训练模型,涵盖数据准备、模型构建、训练过程、模型评估以及模型导出等关键步骤。从一个简单的例子开始,逐步深入,帮助读者掌握TensorFlow模型训练的完整流程。文中将介绍如何使用TensorFlow内置的数据集或自定义数据加载方法,如何选择合适的模型架构和优化器,如何监控训练过程以及如何评估模型性能。此外,还会讲解如何将训练好的模型导出为可部署的格式,例如用于移动设备的tflite格式,方便读者将模型应用到实际场景中。无论你是深度学习的初学者还是有一定经验的开发者,本文都将提供有价值的指导和参考。
在使用TensorFlow训练模型之前,数据获取是关键的第一步。TensorFlow提供了一系列内置的数据集,如MNIST、CIFAR-10等,可以通过tf.keras.datasets
模块轻松加载。
import tensorflow as tf
(train_images, train_labels), (test_images, test_labels) = tf.keras.datasets.mnist.load_data()
数据的处理包括清洗、转换和标准化。在TensorFlow中,tf.data
API是一个强大的工具,可以用于批处理、打乱和缓存数据。
import tensorflow as tf
dataset = tf.data.Dataset.from_tensor_slices(filenames)
# 对每个文件进行解码和预处理
dataset = dataset.map(load_image).batch(32)
在创建模型时,使用TensorFlow的Keras API可以快速构建神经网络。以下是一个简单的全连接网络示例。
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, Flatten
model = Sequential([
Flatten(input_shape=(28, 28)),
Dense(128, activation='relu'),
Dense(10, activation='softmax')
])
TensorFlow内置的数据集是非常方便的工具,可以直接用于模型训练,避免了数据收集和整理的麻烦。
import tensorflow as tf
mnist = tf.keras.datasets.mnist
(x_train, y_train), (x_test, y_test) = mnist.load_data()
tf.data
API不仅可以处理内置数据集,还可以处理自定义数据集。它能有效地处理复杂的数据输入管道。
dataset = tf.data.Dataset.from_tensor_slices((x_train, y_train))
dataset = dataset.shuffle(10000).batch(32)
数据增强有助于提高模型的泛化能力。ImageDataGenerator
可用于图像数据的实时数据增强。
from tensorflow.keras.preprocessing.image import ImageDataGenerator
data_gen = ImageDataGenerator(rotation_range=40, width_shift_range=0.2, height_shift_range=0.2)
标准化是数据处理的基本步骤,确保数据在模型输入时具有相同的尺度。
x_train, x_test = x_train / 255.0, x_test / 255.0
通过tf.data.Dataset.map
中的num_parallel_calls
参数,实现数据的并行处理,加快数据管道的速度。
dataset = dataset.map(preprocess_image, num_parallel_calls=tf.data.AUTOTUNE)
使用cache
方法可以避免在每个epoch都重复读取数据,提升训练速度。
dataset = dataset.cache()
使用Keras的Sequential API定义模型结构,包括输入层、隐藏层和输出层。
model = tf.keras.models.Sequential([
tf.keras.layers.Flatten(input_shape=(28, 28)),
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
编译模型时需指定优化器、损失函数和评估指标。
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
使用fit
方法来训练模型,传入训练数据、批大小和epoch数。
model.fit(x_train, y_train, epochs=5)
自定义模型通常需要自定义的数据集,这需要额外的数据预处理步骤。
import numpy as np
x_custom = np.random.random((1000, 28, 28))
y_custom = np.random.randint(10, size=(1000,))
根据任务需求设计模型结构,可能需要更复杂的层次和激活函数。
model.add(tf.keras.layers.Conv2D(32, (3, 3), activation='relu'))
训练自定义模型时,需不断调试模型参数和结构,观察模型的学习曲线。
history = model.fit(x_custom, y_custom, epochs=10, validation_split=0.2)
使用测试集评估模型性能,查看准确率、损失等指标。
test_loss, test_acc = model.evaluate(x_test, y_test)
优化模型可通过调节学习率、使用正则化、批归一化等技术。
model.add(tf.keras.layers.BatchNormalization())
使用网格搜索或随机搜索方法调整模型超参数,提升模型性能。
from sklearn.model_selection import GridSearchCV
训练完成后,将模型导出为HDF5或SavedModel格式,以便部署。
model.save('my_model.h5')
如需在移动端或边缘设备上部署模型,需将模型转换为TensorFlow Lite格式。
converter = tf.lite.TFLiteConverter.from_saved_model('my_model')
tflite_model = converter.convert()
将转换后的模型部署到目标平台,并进行推理测试。
interpreter = tf.lite.Interpreter(model_content=tflite_model)
interpreter.allocate_tensors()
tf.keras.datasets
模块轻松加载内置数据集,例如MNIST和CIFAR-10。获取到数据后,可以使用tf.data
API进行数据清洗、转换和标准化。以下是一个加载MNIST数据集的示例代码:import tensorflow as tf
(train_images, train_labels), (test_images, test_labels) = tf.keras.datasets.mnist.load_data()
dataset = tf.data.Dataset.from_tensor_slices((train_images, train_labels))
dataset = dataset.shuffle(10000).batch(32)
Sequential
模型来定义输入层、隐藏层和输出层。以下是一个简单的全连接网络示例:from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, Flatten
model = Sequential([
Flatten(input_shape=(28, 28)),
Dense(128, activation='relu'),
Dense(10, activation='softmax')
])
ImageDataGenerator
进行,它提供了图像数据的实时增强功能。这可以提高模型的泛化能力,避免过拟合。以下是一个简单的使用示例:from tensorflow.keras.preprocessing.image import ImageDataGenerator
data_gen = ImageDataGenerator(rotation_range=40, width_shift_range=0.2, height_shift_range=0.2)
model.add(tf.keras.layers.BatchNormalization())
此外,可以使用网格搜索或随机搜索来调整模型的超参数。
model.save('my_model.h5')
converter = tf.lite.TFLiteConverter.from_saved_model('my_model')
tflite_model = converter.convert()
interpreter = tf.lite.Interpreter(model_content=tflite_model)
interpreter.allocate_tensors()