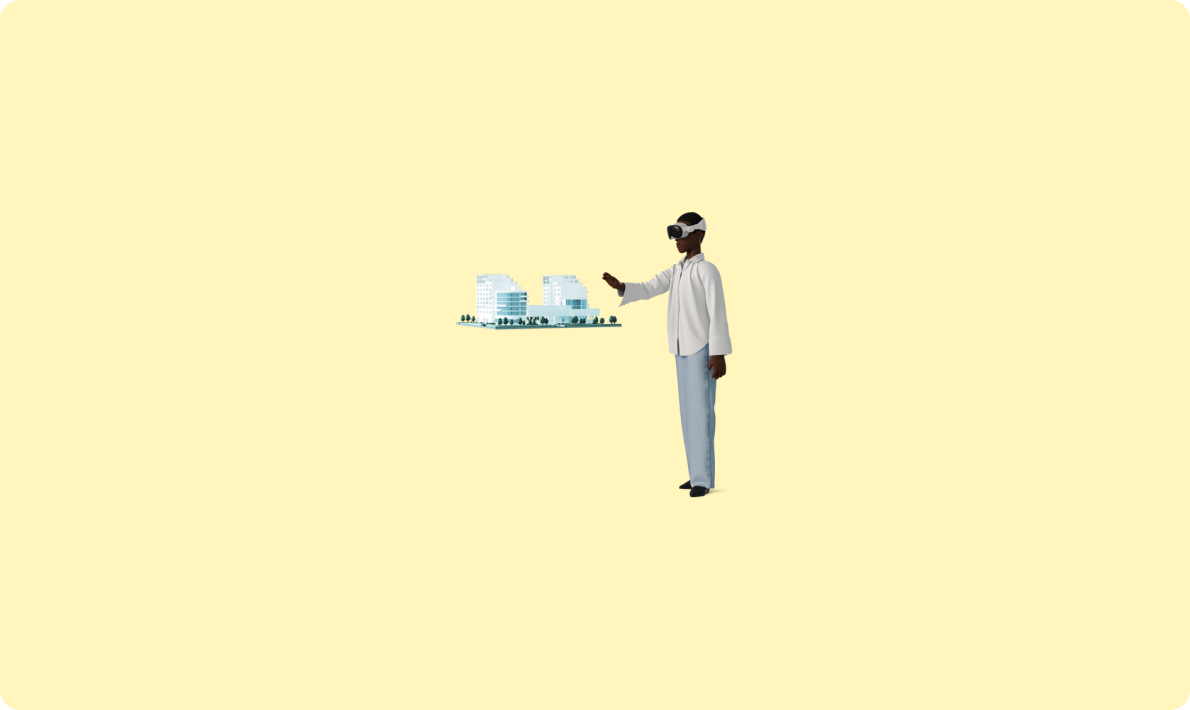
掌握API建模:基本概念和实践
使用 .NET Core 和 Dapper 创建客户端-服务器体系结构涉及使用 .NET Core 和客户端应用程序(例如 Web、桌面或移动设备)构建服务器端 API。Dapper 是一个轻量级的对象关系映射 (ORM) 库,将用于与服务器应用程序中的数据库进行通信。
在此体系结构中,系统分为两个主要实体:请求服务的客户端和提供服务的服务器。REST API 充当向各种客户端(如 Web 浏览器、移动应用程序或其他服务)提供数据的服务器。
1. 创建 .NET Core Web API 项目
打开终端或 Visual Studio,并创建新的 Web API 项目:
dotnet new webapi -n DapperApi
cd DapperApi
2. 安装依赖项
添加 Dapper 和任何必要的 SQL Server 包:
dotnet add package Dapper
dotnet add package Microsoft.Data.SqlClient
3. 配置数据库连接
{
"ConnectionStrings": {
//"DefaultConnection": "Server=localhost;Database=OrderManagement;Trusted_Connection=True;MultipleActiveResultSets=true",
"DefaultConnection": "Server=localhost;Database=OrderManagement;User Id=sa;Password=smicr@123; Encrypt=True;TrustServerCertificate=True;"
}
4. 创建模型
在该文件夹中,为 创建一个模型类 :ModelsProduct
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
}
5. 创建数据库
使用以下 SQL 脚本为 Orders 创建数据库和表。
CREATE DATABASE OrderManagement;
USE OrderManagement;
CREATE TABLE Products
(
Id INT PRIMARY KEY IDENTITY(1,1),
Name NVARCHAR(100),
Price DECIMAL(18,2)
);
6. 使用 Dapper 创建 Repository Layer
在您的解决方案中,创建一个文件夹,然后添加一个接口和一个类以使用 Dapper 处理数据库查询。DataAccess
接口:IProductRepository.cs
public interface IProductRepository
{
Task<IEnumerable<Product>> GetAllProducts();
Task<Product> GetProductById(int id);
Task<int> AddProduct(Product product);
Task<int> UpdateProduct(Product product);
Task<int> DeleteProduct(int id);
}
存储 库:ProductRepository.cs
using Dapper;
using Microsoft.Data.SqlClient;
using Microsoft.Extensions.Configuration;
public class ProductRepository : IProductRepository
{
private readonly DapperContext _dapperContext;
public ProductRepository(DapperContext dapperContext)
{
_dapperContext = dapperContext;
}
public async Task<IEnumerable<Product>> GetAllProducts()
{
using (var connection = _dapperContext.CreateConnection())
{
string sql = "SELECT * FROM Products";
return await connection.QueryAsync<Product>(sql);
}
}
public async Task<Product> GetProductById(int id)
{
using (var connection = _dapperContext.CreateConnection())
{
string sql = "SELECT * FROM Products WHERE Id = @Id";
return await connection.QueryFirstOrDefaultAsync<Product>(sql, new { Id = id });
}
}
public async Task<int> AddProduct(Product product)
{
using (var connection = _dapperContext.CreateConnection())
{
string sql = "INSERT INTO Products (Name, Price) VALUES (@Name, @Price)";
return await connection.ExecuteAsync(sql, product);
}
}
public async Task<int> UpdateProduct(Product product)
{
using (var connection = _dapperContext.CreateConnection())
{
string sql = "UPDATE Products SET Name = @Name, Price = @Price WHERE Id = @Id";
return await connection.ExecuteAsync(sql, product);
}
}
public async Task<int> DeleteProduct(int id)
{
using (var connection = _dapperContext.CreateConnection())
{
string sql = "DELETE FROM Products WHERE Id = @Id";
return await connection.ExecuteAsync(sql, new { Id = id });
}
}
}
7. 在依赖注入中注册仓库
在 中,在 DI 容器中注册 :Program.csProductRepository
using DapperApi.Contacts;
using DapperApi.Data;
using DapperApi.Repositorys;
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
// Add services to the container
builder.Services.AddControllers();
builder.Services.AddSingleton<DapperContext>();
// Configure Dependency Injection for services and repositories
builder.Services.AddScoped<IProductRepository, ProductRepository>();
// Configure Dapper with SQL Server
builder.Services.AddSingleton<IConfiguration>(builder.Configuration);
// Learn more about configuring Swagger/OpenAPI at https://aka.ms/aspnetcore/swashbuckle
builder.Services.AddEndpointsApiExplorer();
builder.Services.AddSwaggerGen();
var app = builder.Build();
// Configure the HTTP request pipeline.
if (app.Environment.IsDevelopment())
{
app.UseSwagger();
app.UseSwaggerUI();
}
app.UseHttpsRedirection();
app.UseAuthorization();
app.MapControllers();
app.Run();
8. 运行应用程序
使用 POST /api/Products
{
"name": "Laptop",
"price": 3000
}
创建控制台应用程序以与 API 交互。
dotnet new console -n ClientApp
cd ClientApp
2. 安装 HttpClient
添加包以与 Web API 交互:System.Net.Http.Json
dotnet add package System.Net.Http.Json
3. 创建客户端代码以与 API 交互
在 中,编写代码以调用 API:Program.cs
// See https://aka.ms/new-console-template for more information
using System.Net.Http.Json;
using System;
using System.Net.Http;
using System.Net.Http.Json;
using System.Threading.Tasks;
Console.WriteLine("Hello, World!");
HttpClient _httpClient = new HttpClient();
string apiUrl = "https://localhost:5001/api/products";
// Get all products
var products = await _httpClient.GetFromJsonAsync<Product[]>(apiUrl);
foreach (var product in products)
{
Console.WriteLine($"{product.Id}: {product.Name} - ${product.Price}");
}
// Add a new product
var newProduct = new Product { Name = "New Product", Price = 19.99M };
var response = await _httpClient.PostAsJsonAsync(apiUrl, newProduct);
if (response.IsSuccessStatusCode)
{
Console.WriteLine("Product added successfully.");
}
// Get a product by id
var productById = await _httpClient.GetFromJsonAsync<Product>($"{apiUrl}/1");
Console.WriteLine($"{productById.Id}: {productById.Name} - ${productById.Price}");
// Update a product
var updateProduct = new Product { Id = 1, Name = "Updated Product", Price = 24.99M };
await _httpClient.PutAsJsonAsync($"{apiUrl}/1", updateProduct);
// Delete a product
await _httpClient.DeleteAsync($"{apiUrl}/2");
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
}
4. 运行客户端
dotnet run
结果
Hello, World!
1: Laptop - $3000.00
Product added successfully.
1: Laptop - $3000.00
文章转自微信公众号@DotNet NB