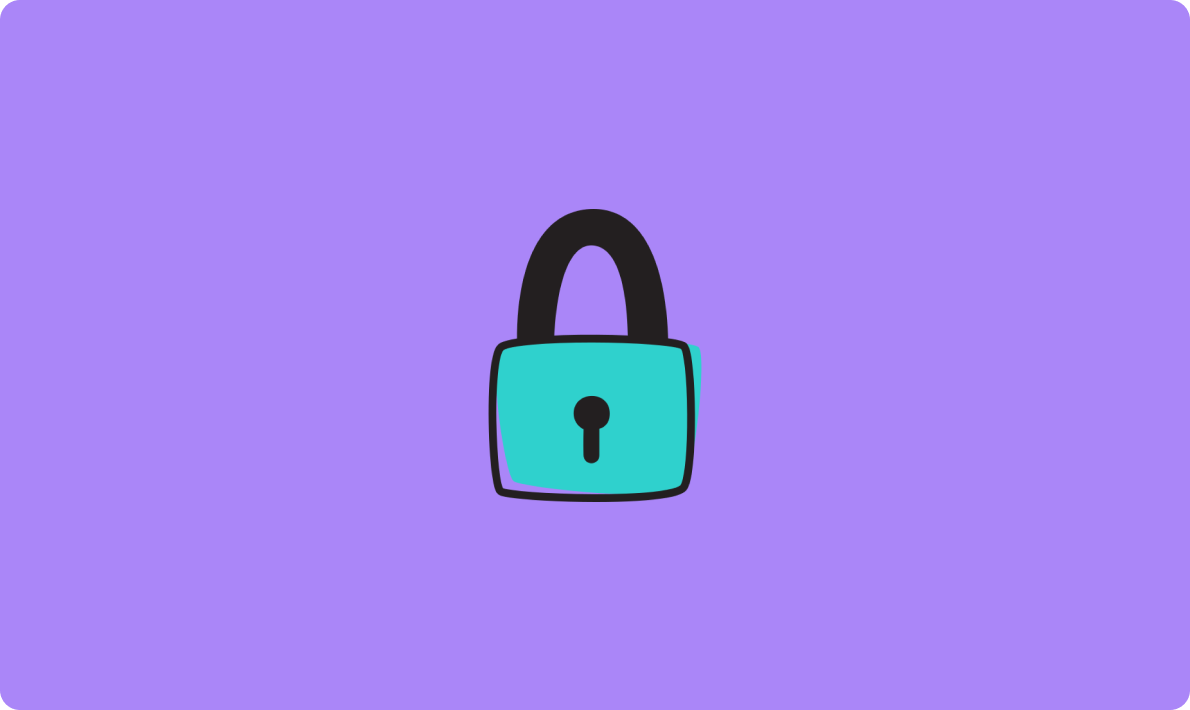
GLIDE 常用提示词:稳定扩散模型的深度解析
在现代图像生成领域,Stable Diffusion已成为一项备受关注的技术。其基于深度学习的文本到图像生成能力,为许多应用场景带来了创新。然而,将Stable Diffusion模型直接集成到Java环境中是一项挑战。本文将详细探讨如何在Java中调用Stable Diffusion API,并提供相应的代码示例和实现步骤。
在Java中直接运行Stable Diffusion模型并不实际,这主要是因为Java不直接支持深度学习模型的运行。然而,我们可以通过调用外部的Python脚本来实现这一功能。以下是一个简单的实现示例。
首先,我们需要确保Python环境中安装了必要的库,如Transformers和PyTorch。这些库将用于加载和运行Stable Diffusion模型。
pip install transformers torch
接下来,创建一个Python脚本stable_diffusion.py
,该脚本将使用Transformers库加载Stable Diffusion模型并处理图像生成请求。
from transformers import StableDiffusionPipeline
def generate_image(prompt):
pipeline = StableDiffusionPipeline.from_pretrained("CompVis/stable-diffusion-v1-4")
image = pipeline(prompt, num_inference_steps=50, guidance_scale=7.5)[0]['sample']
print(f"Generated image data for prompt: {prompt}")
if __name__ == "__main__":
import sys
if len(sys.argv) > 1:
prompt = ' '.join(sys.argv[1:])
generate_image(prompt)
else:
print("Usage: python stable_diffusion.py ")
通过Runtime.getRuntime().exec()
或ProcessBuilder
,我们可以从Java应用程序中执行上述Python脚本。
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class StableDiffusionJava {
public static void main(String[] args) {
if (args.length < 1) {
System.out.println("Usage: java StableDiffusionJava ");
return;
}
String prompt = String.join(" ", args);
String pythonScriptPath = "python stable_diffusion.py";
try {
ProcessBuilder pb = new ProcessBuilder(pythonScriptPath, prompt);
Process p = pb.start();
BufferedReader reader = new BufferedReader(new InputStreamReader(p.getInputStream()));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
int exitCode = p.waitFor();
System.out.println("Exited with error code : " + exitCode);
} catch (IOException | InterruptedException e) {
e.printStackTrace();
}
}
}
在实现过程中,需要注意以下几点:
为了提高系统的鲁棒性和可扩展性,我们可以通过HTTP请求调用运行Stable Diffusion模型的Python Flask服务器。
首先,确保我们已经安装了Flask库和Transformers库。然后,编写Flask服务器代码来处理图像生成请求。
from flask import Flask, request, jsonify
from transformers import StableDiffusionPipeline
from PIL import Image
import io
import base64
app = Flask(__name__)
pipeline = StableDiffusionPipeline.from_pretrained("CompVis/stable-diffusion-v1-4")
@app.route('/generate', methods=['POST'])
def generate_image():
data = request.json
prompt = data.get('prompt', 'A beautiful landscape')
num_inference_steps = data.get('num_inference_steps', 50)
guidance_scale = data.get('guidance_scale', 7.5)
try:
images = pipeline(prompt, num_inference_steps=num_inference_steps, guidance_scale=guidance_scale)
image = images[0]['sample']
buffered = io.BytesIO()
image.save(buffered, format="PNG")
img_str = base64.b64encode(buffered.getvalue()).decode("utf-8")
return jsonify({'image_base64': img_str})
except Exception as e:
return jsonify({'error': str(e)}), 500
if __name__ == '__main__':
app.run(host='0.0.0.0', port=5000)
Java客户端通过HTTP POST请求与Flask服务器通信,接收生成的图像数据。
// StableDiffusionClient.java
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.nio.charset.StandardCharsets;
import java.util.HashMap;
import java.util.Map;
import org.json.JSONObject;
public class StableDiffusionClient {
public static void main(String[] args) {
String urlString = "http://localhost:5000/generate";
Map data = new HashMap();
data.put("prompt", "A colorful sunset over the ocean");
data.put("num_inference_steps", 50);
data.put("guidance_scale", 7.5);
try {
URL url = new URL(urlString);
HttpURLConnection con = (HttpURLConnection) url.openConnection();
con.setRequestMethod("POST");
con.setRequestProperty("Content-Type", "application/json; utf-8");
con.setRequestProperty("Accept", "application/json");
con.setDoOutput(true);
String jsonInputString = new JSONObject(data).toString();
byte[] postData = jsonInputString.getBytes(StandardCharsets.UTF_8);
try (java.io.OutputStream os = con.getOutputStream()) {
os.write(postData);
}
int responseCode = con.getResponseCode();
System.out.println("POST Response Code : " + responseCode);
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
JSONObject jsonObj = new JSONObject(response.toString());
String imageBase64 = jsonObj.getString("image_base64");
System.out.println("Image Base64: " + imageBase64);
} catch (Exception e) {
e.printStackTrace();
}
}
}
在实现过程中,图片链接的使用可以极大地提升用户体验。在生成的图像中,使用图片链接可以让用户直接查看生成的结果,而不必经过繁琐的下载和文件查看过程。
通过这种方式,我们可以在应用程序中嵌入生成的图像,提升可视化效果和用户满意度。
Stable Diffusion是一种基于深度学习的文本到图像生成模型,能够将自然语言描述转换为图像。
可以通过调用外部Python脚本或通过HTTP请求与Python Flask服务器通信来实现。
Flask服务器提供了更灵活和可扩展的解决方案,便于Java和Python应用程序独立运行并通过网络通信。
需要对用户输入进行严格验证,以防止潜在的安全漏洞,如代码注入攻击。
可以通过使用更高效的模型、调整推理步数和引导比例等参数来优化性能。