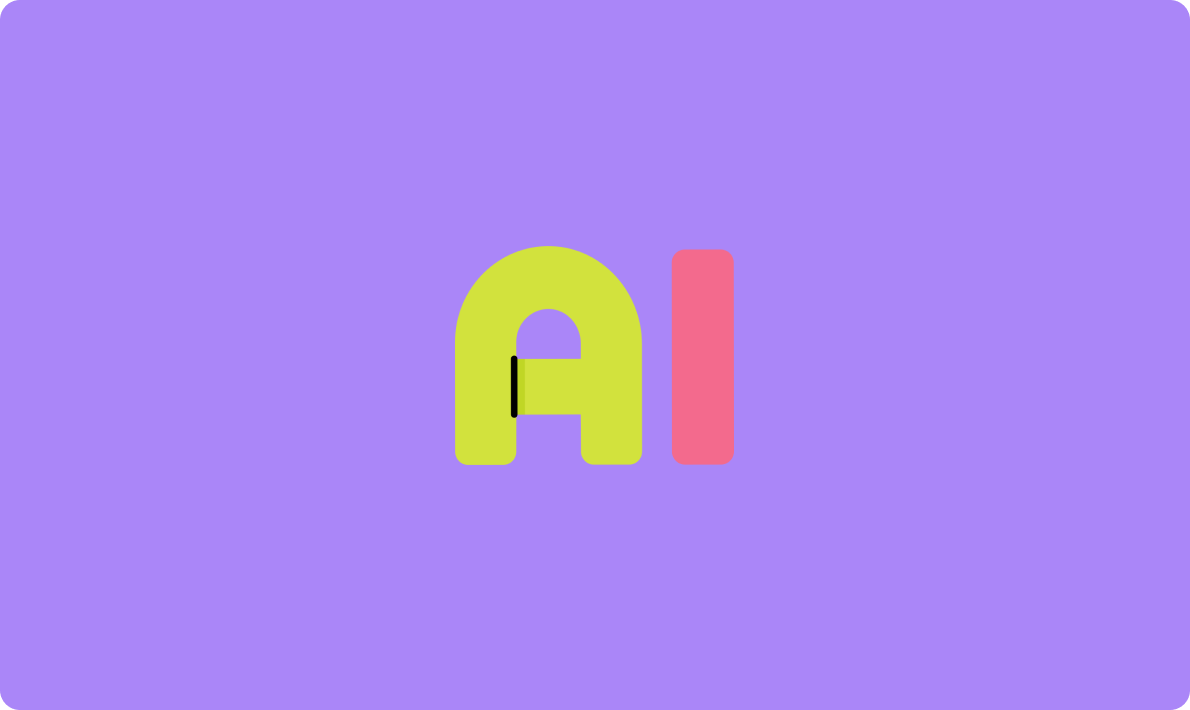
DeepSeek Janus-Pro 应用代码与图片链接实践
在技术的不断发展中,利用Java语言访问即梦AI API,实现从网络小说内容抓取到生成微信公众平台文章,已经成为一种创新的内容创作方式。本文将详细介绍这一过程的实现步骤,涵盖网络小说抓取、AI配图生成、以及微信公众号文章的构建。
在内容创作的第一步,我们需要从网络小说平台抓取所需内容。Java语言提供了丰富的库可以实现这一功能,其中,Jsoup 是一款强大的 HTML 解析器,能够方便地抓取网页内容。
Jsoup 是一个 Java 的 HTML 解析库,可以直接从 URL、文件或字符串中解析 HTML。它提供了非常方便的方法来提取和操作数据。
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import java.io.IOException;
public class NovelScraper {
public static void main(String[] args) {
String url = "http://example.com/novel/chapter1"; // 替换为实际的小说章节URL
try {
Document document = Jsoup.connect(url).get();
Element contentElement = document.getElementById("content");
String content = contentElement.text();
System.out.println(content);
} catch (IOException e) {
e.printStackTrace();
}
}
}
以上代码展示了如何使用Jsoup抓取指定URL的内容,并从中提取文本信息。这一过程可以快速获取小说章节的文字内容,为后续的AI配图和文章生成奠定基础。
在获取到小说内容后,下一步是使用即梦AI的API,根据文本内容生成相应的图片。这些图片将在最终生成的微信公众号文章中使用,提升文章的视觉吸引力。
即梦AI提供了一个RESTful API,通过该API可以根据内容生成图片。下面是一个简单的Java实现示例。
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
public class ImageGenerator {
public static void main(String[] args) {
String apiUrl = "http://api.jimengai.com/generate-image"; // 替换为实际的API URL
String prompt = "A scene from the novel"; // 根据小说内容生成提示
try {
URL url = new URL(apiUrl);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("POST");
connection.setRequestProperty("Content-Type", "application/json; utf-8");
connection.setRequestProperty("Accept", "application/json");
connection.setDoOutput(true);
String jsonInputString = "{"prompt":"" + prompt + ""}";
try(OutputStream os = connection.getOutputStream()) {
byte[] input = jsonInputString.getBytes("utf-8");
os.write(input, 0, input.length);
}
int responseCode = connection.getResponseCode();
System.out.println("Response Code: " + responseCode);
// 处理响应,保存图片到本地或直接使用
} catch (Exception e) {
e.printStackTrace();
}
}
}
通过上述代码,可以将文本提示发送给即梦AI的API,并接收生成的图片。随后,可以根据需要将图片保存到本地或直接获取其URL,以供后续使用。
完成内容抓取与配图后,最后一步是将这些信息整合,生成格式化的微信公众号文章。通过Java程序,可以轻松将文字内容和图像整合成HTML格式,便于发布。
通过简单的字符串操作,我们可以将抓取的内容和生成的图片组合成微信文章的HTML格式。
public class WeChatArticleGenerator {
public static void main(String[] args) {
String title = "Chapter 1 of the Novel";
String content = "Here is the content of the chapter..."; // 从NovelScraper获取
String imageUrl = "http://example.com/image.jpg"; // 从ImageGenerator获取
StringBuilder article = new StringBuilder();
article.append("").append(title).append("
");
article.append("
");
article.append("").append(content).append("
");
String weChatArticle = article.toString();
System.out.println(weChatArticle);
// 将生成的文章发布到微信公众号
}
}
通过这种方式,可以生成完整的文章内容,并可以进一步使用微信公众平台的API将其发布。
在整个实现过程中,API调用是关键的一环。在此过程中,需特别注意API请求的格式和参数设置,以确保成功获取响应。
以即梦AI的API为例,需确保请求的Content-Type为application/json,并在请求体中包含正确的JSON格式的提示信息。
此外,Java也可以用来调用其他AI服务,例如OpenAI API。下面是一个使用Hutool HTTP工具类库进行API调用的例子。
import cn.hutool.http.HttpRequest;
import cn.hutool.http.HttpResponse;
import cn.hutool.http.HttpUtil;
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONObject;
import java.util.HashMap;
public class OpenAiUitl {
public static JSONObject execute(String url, String key, String value) {
HashMap headers = new HashMap();
headers.put("Content-Type", "application/json");
headers.put("Authorization", "Bearer " + key);
HttpRequest post = HttpUtil.createPost(url);
post.addHeaders(headers);
JSONObject data = new JSONObject();
data.put("model", "gpt-3.5-turbo");
JSONArray array = new JSONArray();
JSONObject messages = new JSONObject();
messages.put("role", "user");
messages.put("content", value);
array.add(messages);
data.put("messages", array);
post.body(data.toJSONString());
HttpResponse execute = post.execute();
return JSONObject.parseObject(execute.body());
}
public static void main(String[] args) {
JSONObject java = execute("https://api.openai.com/v1/chat/completions",
"你的key", "java");
System.out.println(java);
}
}
通过这种方式,我们可以轻松地将Java应用程序与OpenAI API集成,实现创新的人工智能应用。
通过本文所述的步骤,您可以利用Java语言实现完整的内容生成流程,从网络小说抓取到AI配图,再到微信公众平台文章的构建,提升内容创作的效率和质量。