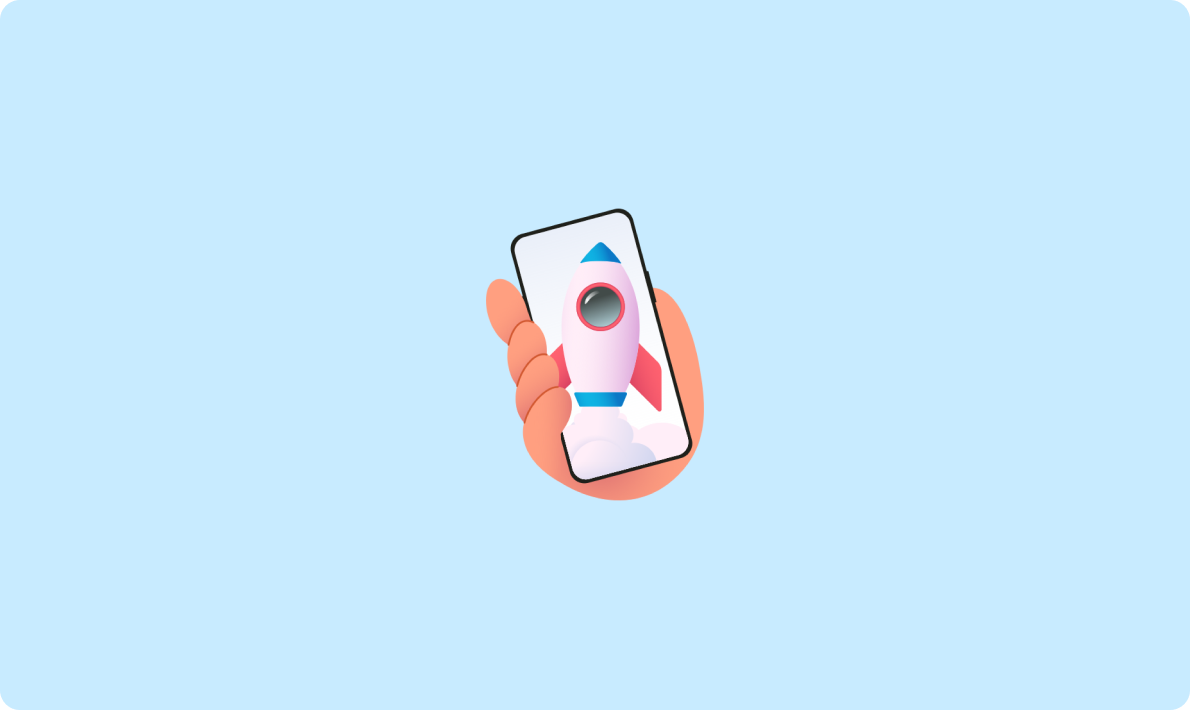
Stable Diffusion Agent 开发:技术解析与应用前景
本文将深入探讨如何在Java中调用FLUX.1-dev API以实现AI绘画功能。FLUX作为AI绘画界的新星,提供了强大的API接口,使开发者无需购买昂贵的显卡即可快速部署和调用AI模型。此外,本文还将介绍如何使用Java进行响应式编程,创建Flux和Mono对象,以及在程序中实现流的动态生成、异常处理和背压控制等功能。通过这些技术的结合,开发者可以轻松构建高效、灵活的AI应用程序。
FLUX.1-dev 是一个强大的开源 AI 绘画模型,可以在线体验,用户无需本地显卡即可使用。提供了两个版本,Schnell 和 Dev,分别在生成速度和质量上各有优势。想要体验的用户可以通过 硅基流动的注册页面 注册,获得免费的体验额度。
在使用 FLUX.1-dev 进行绘画时,建议使用英文提示词以取得更好的效果,例如:
Prompt: a young artist sitting in front of a canvas, focused on painting, surrounded by colorful paints and brushes, sunlight streaming through the window onto him.
FLUX.1-dev 还提供 API 调用功能,方便开发者将其集成到应用中。API 文档可以在这里 找到。
import requests
url = "https://api.siliconflow.cn/v1/black-forest-labs/FLUX.1-schnell/text-to-image"
payload = {
"prompt": "an island near sea, with seagulls, moon shining over the sea, light house, boats in the background, fish flying over the sea",
"image_size": "1024x1024",
"batch_size": 1,
"num_inference_steps": 20,
"guidance_scale": 7.5
}
headers = {
"accept": "application/json",
"content-type": "application/json",
"Authorization": "Bearer your_api_key"
}
response = requests.post(url, json=payload, headers=headers)
print(response.text)
在 Java 中,使用 Flux 和 Mono 可以轻松创建响应式流。使用 Flux.just()
和 Mono.just()
方法,可以从简单的数据集合中创建流。
Flux.just(1, 2, 3, 4).subscribe(System.out::println);
Mono.just("Hello").subscribe(System.out::println);
Flux.fromArray()
方法可以从数组中创建 Flux 流,适用于已知大小的数据集。
Flux.fromArray(new Integer[]{1, 2, 3, 4}).subscribe(System.out::println);
Flux 和 Mono 可以相互转换。使用 collectList()
可以将 Flux 转换为 Mono,反之亦然。
Flux flux = Flux.just(1, 2, 3);
Mono<List> mono = flux.collectList();
mono.subscribe(System.out::println);
通过 Flux.fromIterable()
方法,可以从任何实现了 Iterable 接口的对象中创建 Flux 流。这种方法非常灵活,适用于多种数据源。
List list = Arrays.asList(1, 2, 3, 4);
Flux.fromIterable(list).subscribe(System.out::println);
当使用 Iterable 对象创建 Flux 后,可以动态添加元素到 Iterable 中,Flux 将自动包含这些新元素。
ArrayList list = new ArrayList(Arrays.asList(1, 2, 3));
Flux flux = Flux.fromIterable(list);
list.add(4);
flux.subscribe(System.out::println);
即使 Iterable 对象为空,Flux.fromIterable()
也能创建一个空的 Flux 而不会抛出异常。
List emptyList = Collections.emptyList();
Flux.fromIterable(emptyList).subscribe(System.out::println);
通过 Java 的 Stream API,可以将数据流转换为 Flux 流。Flux.fromStream()
是一个简单而强大的工具。
Flux.fromStream(Stream.of(1, 2, 3, 4)).subscribe(System.out::println);
Flux.range()
方法可以生成一个整数序列的 Flux 流,适用于需要生成特定范围数字的场景。
Flux.range(0, 10).subscribe(System.out::println);
可以将 Stream 和 Range 结合起来使用,进一步扩展 Flux 的功能。
Stream stream = Stream.iterate(0, n -> n + 1).limit(10);
Flux.fromStream(stream).subscribe(System.out::println);
在开发过程中,处理异常是不可或缺的一部分。可以直接创建一个包含异常的 Flux 或 Mono。
Flux.error(new RuntimeException("Error occurred")).subscribe(System.out::println, System.err::println);
Mono.error(new RuntimeException("Error occurred")).subscribe(System.out::println, System.err::println);
通过 doOnError()
方法,可以在异常发生时执行特定的操作,比如记录日志。
Mono.just("start")
.concatWith(Mono.error(new RuntimeException("Exception")))
.doOnError(error -> System.out.println("Caught: " + error))
.subscribe(System.out::println);
当异常发生时,可以通过 onErrorResume()
方法生成一个新的流,作为替代方案。
Flux.just(1, 2, 3)
.concatWith(Mono.error(new RuntimeException("Error")))
.onErrorResume(e -> Flux.just(4, 5, 6))
.subscribe(System.out::println);
使用 merge()
方法可以将多个 Flux 流合并到一起,合并后的流会按时间顺序输出所有的元素。
Flux.merge(Flux.just(1, 2), Flux.just(3, 4)).subscribe(System.out::println);
filter()
方法用于从 Flux 流中过滤掉不符合条件的元素,只保留满足条件的元素。
Flux.range(1, 10).filter(i -> i % 2 == 0).subscribe(System.out::println);
zipWith()
方法可以将两个 Flux 的元素一一配对,并对配对的元素进行处理。
Flux.just(1, 2).zipWith(Flux.just("A", "B"), (num, letter) -> num + letter).subscribe(System.out::println);
在处理响应式流时,背压机制用于控制数据流速,以避免资源消耗过大。通过 BaseSubscriber
可以自定义背压策略。
Flux.range(1, 10).subscribe(new BaseSubscriber() {
@Override
protected void hookOnSubscribe(Subscription subscription) {
request(2);
}
@Override
protected void hookOnNext(Integer value) {
System.out.println(value);
if (value == 2) {
cancel();
}
}
});
在 subscribe()
方法中,可以指定每次请求的元素数量,从而控制流的消费速率。
Flux.range(1, 100).limitRate(10).subscribe(System.out::println);
通过实现 Subscriber
接口,可以完全掌控数据流的请求和消费逻辑。
Flux.range(1, 10).subscribe(new Subscriber() {
private Subscription subscription;
private int count = 0;
@Override
public void onSubscribe(Subscription s) {
this.subscription = s;
subscription.request(1);
}
@Override
public void onNext(Integer integer) {
System.out.println(integer);
if (++count >= 2) {
subscription.request(1);
count = 0;
}
}
@Override
public void onError(Throwable t) {
t.printStackTrace();
}
@Override
public void onComplete() {
System.out.println("Done");
}
});
a young artist sitting in front of a canvas, focused on painting, surrounded by colorful paints and brushes, sunlight streaming through the window onto him.
这种详细的描述有助于模型生成更符合预期的图像。import requests
url = "https://api.siliconflow.cn/v1/black-forest-labs/FLUX.1-schnell/text-to-image"
payload = {
"prompt": "an island near sea, with seagulls, moon shining over the sea, light house, boats in the background, fish flying over the sea",
"image_size": "1024x1024",
"batch_size": 1,
"num_inference_steps": 20,
"guidance_scale": 7.5
}
headers = {
"accept": "application/json",
"content-type": "application/json",
"Authorization": "Bearer your_api_key"
}
response = requests.post(url, json=payload, headers=headers)
print(response.text)
Flux.just()
和 Mono.just()
可以轻松创建响应式流。Flux
用于处理多个元素的数据流,而 Mono
适用于单个元素或可选的场景。例如:Flux.just(1, 2, 3, 4).subscribe(System.out::println);
Mono.just("Hello").subscribe(System.out::println);
doOnError()
方法来捕获和处理异常事件,或者使用 onErrorResume()
为异常提供替代的数据流。例如:Mono.just("start")
.concatWith(Mono.error(new RuntimeException("Exception")))
.doOnError(error -> System.out.println("Caught: " + error))
.subscribe(System.out::println);
通过这种方式,开发者能够更好地控制和处理流中的异常情况。