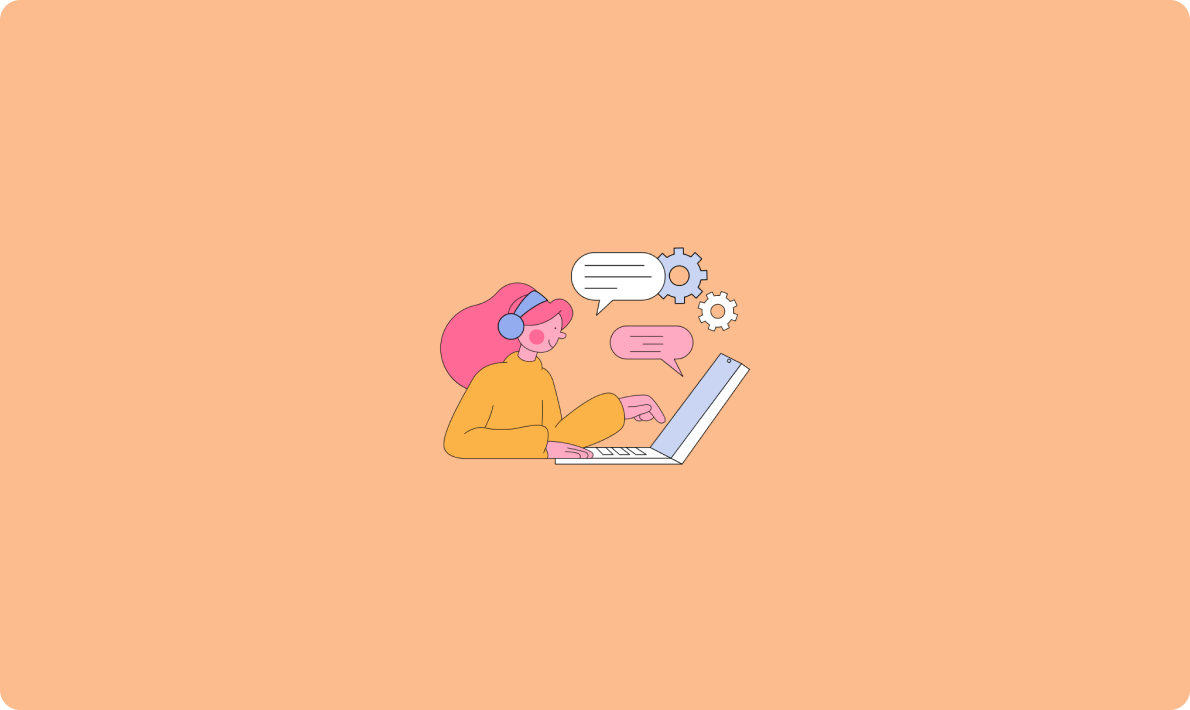
文心一言写代码:代码生成力的探索
LangChain 是一个强大的开发框架,旨在通过集成多个工具和语言模型来构建智能代理。本文将重点介绍如何在 LangChain 中开发 GLM-4 Agent,涵盖从基础组件到高级应用的各个方面。
GLM-4 Agent 是一种通过集成大语言模型和多种工具,能够自主理解、规划决策并执行复杂任务的智能体。它的核心优势在于基于 LLM(大语言模型)的推理能力,能够动态决定操作步骤和工具使用顺序。与传统的 Chain 相比,Agent 更灵活,因为它不像 Chain 那样被硬编码为一系列固定的操作。
GLM-4 Agent 由多种核心组件构成,包括 Model IO、Retrieval、Chain 和 Agent。Agent 是其中最重要的部分,因为它负责将大脑(LLM)与外部工具结合,从而提高大脑的知识获取能力。这种结合使得智能体可以利用外部资源更有效地完成任务。
LangChain 内置了多种 Agent 类型,每种类型都有不同的推理提示风格、编码输入方式和解析输出方式。例如,OpenAI Tools 和 OpenAI Functions 主要为 OpenAI 模型设计,支持对话历史、多个输入参数工具和并行函数调用。Structured Chat 则支持多个输入参数工具的使用,适合需要复杂输入处理的场景。
开发 GLM-4 Agent 涉及多个步骤,包括定义工具、创建 Agent 和 Agent 执行器。以下是详细的开发流程。
在开发过程中,我们可以创建自定义工具来完成特定的计算任务。下面是创建乘法、加法和指数工具的示例代码。
from langchain_core.tools import tool
@tool
def multiply(first_int: int, second_int: int) -> int:
"""Multiply two integers together."""
return first_int * second_int
@tool
def add(first_int: int, second_int: int) -> int:
"Add two integers."
return first_int + second_int
@tool
def exponentiate(base: int, exponent: int) -> int:
"Exponentiate the base to the exponent power."
return base**exponent
LangChain 提供了多个内置工具,例如 Python 代码解析器、Duckduckgo 搜索引擎和维基百科查询工具。我们可以通过以下代码引入这些工具。
from langchain_experimental.tools import PythonREPLTool
pythonREPLTool = PythonREPLTool()
from langchain.tools import WikipediaQueryRun
from langchain_community.utilities import WikipediaAPIWrapper
wikipedia = WikipediaQueryRun(api_wrapper=WikipediaAPIWrapper())
from langchain.tools import DuckDuckGoSearchRun
search = DuckDuckGoSearchRun()
ReAct 是一种框架,允许 LLM 通过逻辑推理和一系列行动达成目标。它协调了 LLM 与外部信息和功能的交互。在 LangChain 中,ReAct 是 Agent 的基础架构,帮助智能体做出合理的决策。
ReAct 提示词模板包括系统消息、消息占位符和人类消息。系统消息定义了工具的使用方式,消息占位符保存对话历史,人类消息包含用户输入的问题。
================================ System Message ================================
Respond to the human as helpfully and accurately as possible. You have access to the following tools:
{tools}
Use a json blob to specify a tool by providing an action key (tool name) and an action_input key (tool input).
Valid "action" values: "Final Answer" or {tool_names}
Provide only ONE action per $JSON_BLOB, as shown:
{
"action": $TOOL_NAME,
"action_input": $INPUT
}
Follow this format:
Question: input question to answer
Thought: consider previous and subsequent steps
Action:
$JSON_BLOB
Observation: action result
... (repeat Thought/Action/Observation N times)
Thought: I know what to respond
Action:
{
"action": "Final Answer",
"action_input": "Final response to human"
}
Begin! Reminder to ALWAYS respond with a valid json blob of a single action. Use tools if necessary. Respond directly if appropriate. Format is Action:$JSON_BLOB
then Observation
================================= Messages Placeholder ==============================
{chat_history}
================================ Human Message =================================
{input}
{agent_scratchpad}
(reminder to respond in a JSON blob no matter what)
## 开发 Agent 执行器
Agent 执行器是 Agent 的运行时对象,负责调用 Agent 智能体,执行它选择的操作,并将结果传回 Agent。以下是创建 Agent 执行器的代码。
```python
from langchain.agents import AgentExecutor
agent_executor = AgentExecutor(agent=agent, tools=tools, handle_parsing_errors=True, verbose=True)
@tool
装饰器,将其注册为 LangChain 的工具。通过以上步骤,您可以成功开发出一个功能强大的 GLM-4 Agent,实现智能任务处理和复杂决策。