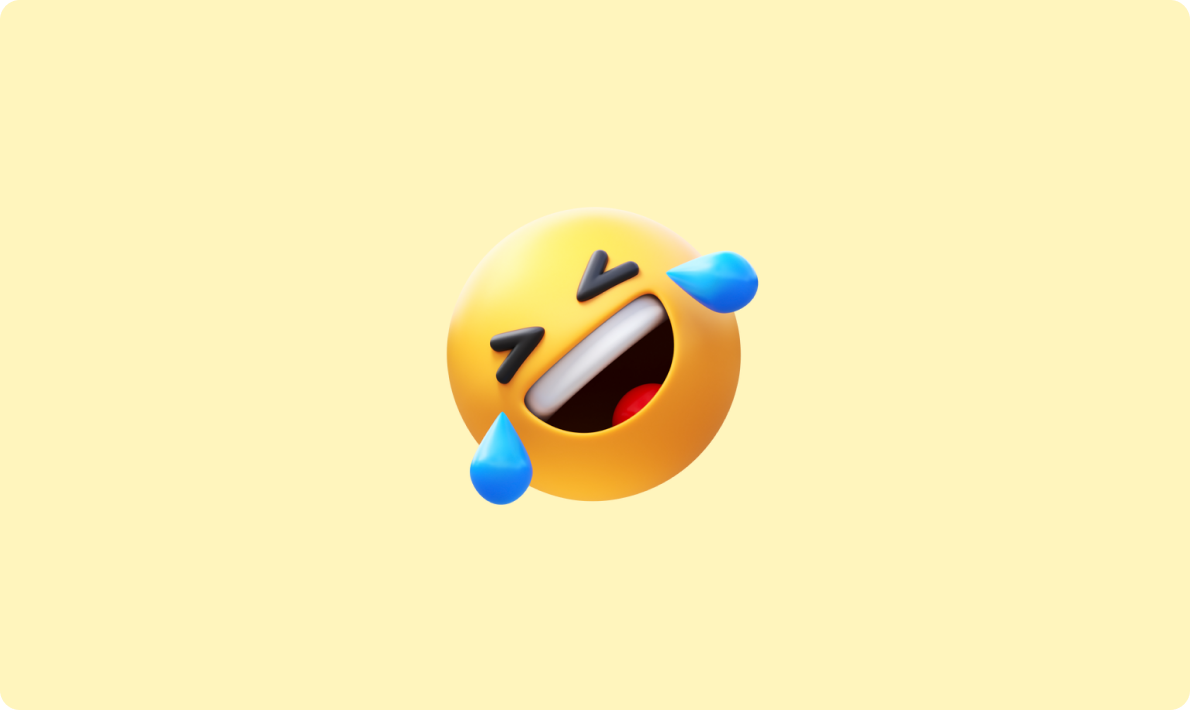
Python实现动图生成:轻松创建自定义表情包
开发者朋友们,大家好!如果您曾经在处理混乱的API响应时感到困惑,那么您来对地方了。今天,我们将探讨在Spring Boot中构建和处理API响应的最佳实践。遵循这些方法,您最终将得到一个清晰且可行的计划,这将使您的API响应更加清晰、一致,并提升用户体验。
一个结构良好的API响应具有以下优点:
结构良好的 API 响应应该是:
首先创建一个所有 API 都遵循的标准响应格式。以下是一个简单有效的格式:
public class ApiResponse<T> {
private boolean success;
private String message;
private T data;
private List<String> errors;
private int errorCode;
private long timestamp; // Adding timestamp for tracking
private String path; // Adding path to identify the API endpoint
// Constructors, getters, and setters
}
boolean
2.消息 :
String
3.数据:
T
4.错误 :
List<String>
5. 错误代码 :
int
6.时间戳 :
long
7.路径 :
String
让我们创建实用的方法来生成响应,以保持不重复。这样做既可以确保一致性,又能减少样板代码。
public class ResponseUtil {
public static <T> ApiResponse<T> success(T data, String message, String path) {
ApiResponse<T> response = new ApiResponse<>();
response.setSuccess(true);
response.setMessage(message);
response.setData(data);
response.setErrors(null);
response.setErrorCode(0); // No error
response.setTimestamp(System.currentTimeMillis());
response.setPath(path);
return response;
}
public static <T> ApiResponse<T> error(List<String> errors, String message, int errorCode, String path) {
ApiResponse<T> response = new ApiResponse<>();
response.setSuccess(false);
response.setMessage(message);
response.setData(null);
response.setErrors(errors);
response.setErrorCode(errorCode);
response.setTimestamp(System.currentTimeMillis());
response.setPath(path);
return response;
}
public static <T> ApiResponse<T> error(String error, String message, int errorCode, String path) {
return error(Arrays.asList(error), message, errorCode, path);
}
}
我们可以使用 @ControllerAdvice 和 @ExceptionHandler 注解来全局处理异常,确保捕获任何未处理的错误并以标准响应格式返回。
@RestControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public ResponseEntity<ApiResponse<Void>> handleException(HttpServletRequest request, Exception ex) {
List<String> errors = Arrays.asList(ex.getMessage());
ApiResponse<Void> response = ResponseUtil.error(errors, "An error occurred", 1000, request.getRequestURI()); // General error
return new ResponseEntity<>(response, HttpStatus.INTERNAL_SERVER_ERROR);
}
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<ApiResponse<Void>> handleResourceNotFoundException(HttpServletRequest request, ResourceNotFoundException ex) {
ApiResponse<Void> response = ResponseUtil.error(ex.getMessage(), "Resource not found", 1001, request.getRequestURI()); // Resource not found error
return new ResponseEntity<>(response, HttpStatus.NOT_FOUND);
}
@ExceptionHandler(ValidationException.class)
public ResponseEntity<ApiResponse<Void>> handleValidationException(HttpServletRequest request, ValidationException ex) {
ApiResponse<Void> response = ResponseUtil.error(ex.getErrors(), "Validation failed", 1002, request.getRequestURI()); // Validation error
return new ResponseEntity<>(response, HttpStatus.BAD_REQUEST);
}
// Handle other specific exceptions similarly
}
现在,让我们在示例控制器中使用标准化响应结构。
@RestController
@RequestMapping("/api/products")
public class ProductController {
@GetMapping("/{id}")
public ResponseEntity<ApiResponse<Product>> getProductById(@PathVariable Long id, HttpServletRequest request) {
// Fetch product by id (dummy code)
Product product = productService.findById(id);
if (product == null) {
throw new ResourceNotFoundException("Product not found with id " + id);
}
ApiResponse<Product> response = ResponseUtil.success(product, "Product fetched successfully", request.getRequestURI());
return new ResponseEntity<>(response, HttpStatus.OK);
}
@PostMapping
public ResponseEntity<ApiResponse<Product>> createProduct(@RequestBody Product product, HttpServletRequest request) {
// Create new product (dummy code)
Product createdProduct = productService.save(product);
ApiResponse<Product> response = ResponseUtil.success(createdProduct, "Product created successfully", request.getRequestURI());
return new ResponseEntity<>(response, HttpStatus.CREATED);
}
// More endpoints...
}
以下是您可能使用的常见错误代码的快速参考(这些只是示例,您可以根据您的项目进行自定义):
通过标准化错误代码,您可以简化跨应用程序不同层处理错误的过程,从而更轻松地管理和调试问题。这些错误代码可以在前端和后端维护,以确保一致的错误处理并向用户提供有意义的反馈。
在Spring Boot中,实施一种清晰、一致的处理API响应的方法,不仅能使您的API更加简洁和易于维护,而且会让您的前端团队(甚至未来的您)感激不尽。
原文链接:在 Spring Boot 中构建 API 响应的最佳方法 |作者 Rabinarayan Patra |来自 ThoughtClan 的见解 |8 月, 2024 |中等 (medium.com)