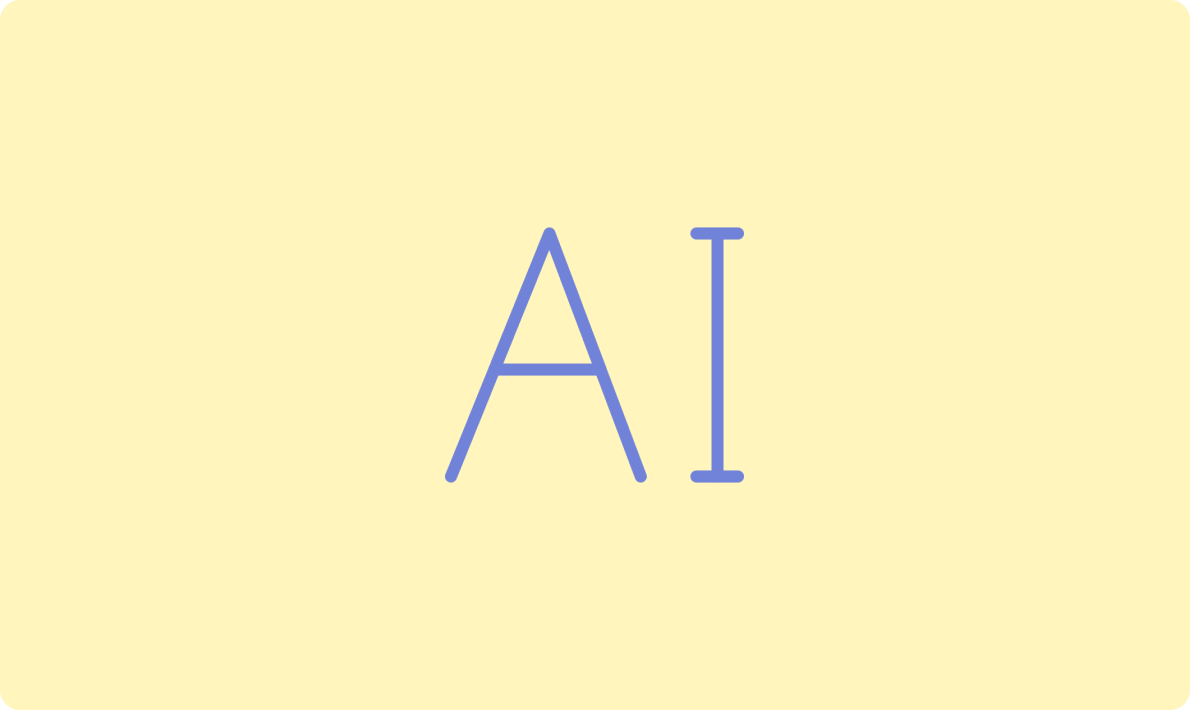
14个文本转图像AI API
AWS Gateway 是一款功能强大的工具,可用于构建可扩展的 API,以满足现代 Web 和移动应用程序的需求。借助 AWS Gateway,您可以创建 RESTful API,向开发人员公开您的数据和业务逻辑,然后开发人员可以构建使用您的 API 的丰富交互式应用程序。
REST API 是构建可扩展分布式 Web 应用程序的行业标准。借助 AWS Gateway,您可以轻松构建支持 GET 和 POST 方法以及复杂查询参数的 REST API。您还可以添加对其他 HTTP 方法(例如 PUT、DELETE 和 HEAD)的支持。
使用 AWS Gateway,您可以快速创建安全且强大的 API。您还可以使用它以最少的努力将代码部署到生产环境。此外,AWS Gateway 允许与其他 AWS 服务(例如 S3 和 DynamoDB)无缝集成,使您能够轻松地向 API 添加复杂的功能。
在使用 AWS Gateway 构建 RESTful API 之前,您应该做好以下准备:
import json
# Example data
data = {
"items": [
{"id": 1, "name": "Item 1", "price": 10.99},
{"id": 2, "name": "Item 2", "price": 15.99},
{"id": 3, "name": "Item 3", "price": 20.99},
]
}
def lambda_handler(event, context):
# Determine the HTTP method of the request
http_method = event["httpMethod"]
# Handle GET request
if http_method == "GET":
# Return the data in the response
response = {
"statusCode": 200,
"body": json.dumps(data)
}
return response
# Handle POST request
elif http_method == "POST":
# Retrieve the request's body and parse it as JSON
body = json.loads(event["body"])
# Add the received data to the example data
data["items"].append(body)
# Return the updated data in the response
response = {
"statusCode": 200,
"body": json.dumps(data)
}
return response
# Handle PUT request
elif http_method == "PUT":
# Retrieve the request's body and parse it as JSON
body = json.loads(event["body"])
# Update the example data with the received data
for item in data["items"]:
if item["id"] == body["id"]:
item.update(body)
break
# Return the updated data in the response
response = {
"statusCode": 200,
"body": json.dumps(data)
}
return response
# Handle DELETE request
elif http_method == "DELETE":
# Retrieve the request's body and parse it as JSON
body = json.loads(event["body"])
# Find the item with the specified id in the example data
for i, item in enumerate(data["items"]):
if item["id"] == body["id"]:
# Remove the item from the example data
del data["items"][i]
break
# Return the updated data in the response
response = {
"statusCode": 200,
"body": json.dumps(data)
}
return response
else:
# Return an error message for unsupported methods
response = {
"statusCode": 405,
"body": json.dumps({"error": "Method not allowed"})
}
return response
此代码定义了一个 Lambda 函数,lambda_handler
用于处理针对某些数据的不同类型的 HTTP 请求(GET
、POST
、PUT
、DELETE
)。数据是一个包含项目数组的对象,每个项目都有一个 ID、名称和价格。
当调用该函数时,它首先从事件对象中确定请求的 HTTP 方法。然后它相应地处理请求:
通过单击“操作”并选择“部署 API”来部署 API。
选择部署阶段(例如“prod”或“test”)并点击“Deploy”。使用生成的 API 端点向您的 API 发出请求。
现在,我们的 API 已启动并运行。您可以通过 Postman 发送测试 HTTP 请求。通过向您的 发送请求invoke URL
,您应该会看到200 OK
状态代码。对于此测试,传入请求不需要请求正文。
这样,我们就使用 AWS Lambda 和 Python 创建了一个简单的 RESTful API。此代码可以作为为您的应用程序创建更复杂 API 的基础。在继续开发 API 时,您可能需要考虑实施安全措施,例如 API 密钥、与 API 网关集成,监控 API 的使用情况或通过以下方式创造收入API 货币化。
原文地址:https://www.moesif.com/blog/technical/api-development/Building-Rest-API-With-AWS-Gateway-And-Python/