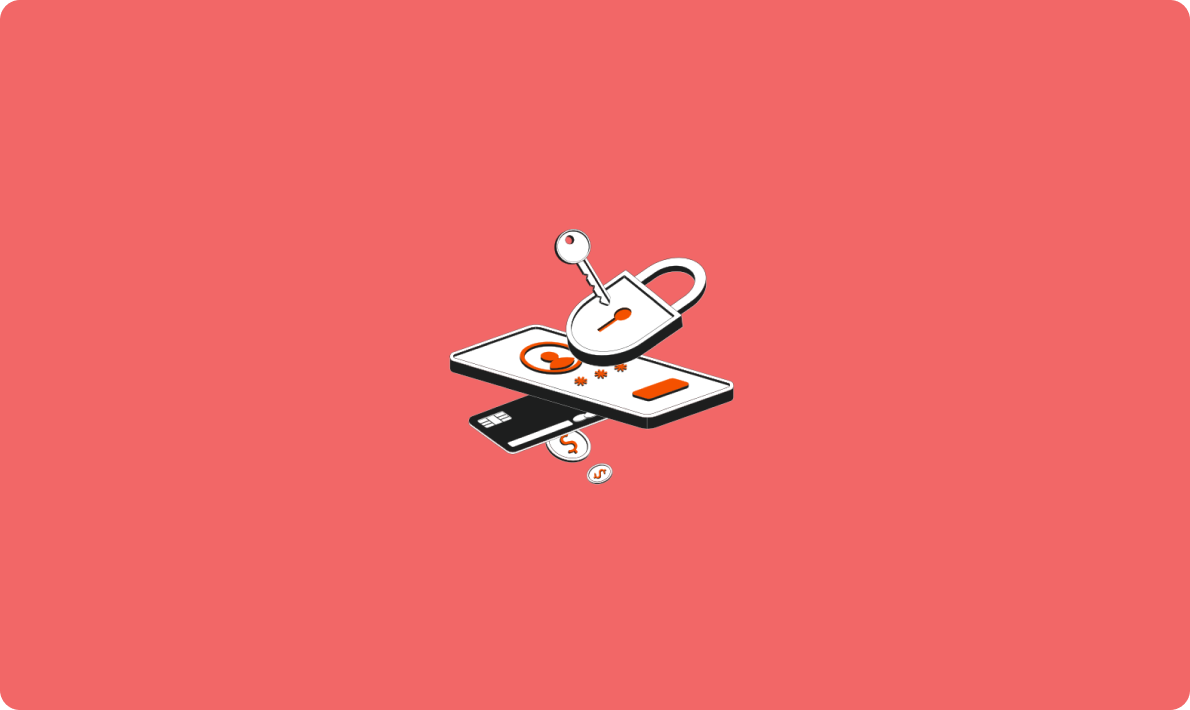
2024年在线市场平台的11大最佳支付解决方案
构建实时应用程序在现代 Web 开发中变得至关重要,尤其是对于通知、聊天系统和实时更新等功能。SignalR 是一个强大的 ASP.NET 库,支持服务器端代码和客户端 Web 应用程序之间的无缝实时通信。https://www.iwmyx.cn/netcoresignalr.html
这里去掉“使用控制器”来构建最小API服务。
最后点击【创建】。
使用 NuGet 包管理器 UI 通过搜索“SignalR”,找到“Microsoft.AspNetCore.SignalR”安装
当然,也可以通过NuGet 包管理器控制台来通过命令安装:
Install-Package Microsoft.AspNetCore.SignalR
builder.Services.AddSignalR();
using Microsoft.AspNetCore.SignalR;
namespace SignalRApi
{
public class SignalRHub : Hub
{
/// <summary>
/// 发布学生成绩
/// </summary>
/// <param name="name">学生姓名</param>
/// <param name="score">得分</param>
/// <param name="msg">备注</param>
/// <returns></returns>
public async Task SendMessage(string name, int score, string msg)
{
await Clients.All.SendAsync("ReceiveScore", name, score, msg);
}
public override async Task OnConnectedAsync()
{
string connectionId = Context.ConnectionId;
await base.OnConnectedAsync();
}
}
}
具体业务逻辑代码:
using Microsoft.AspNetCore.SignalR;
namespace SignalRApi
{
public class WorkerService : BackgroundService
{
private readonly IHubContext<SignalRHub> _signalRHub;
public WorkerService(IHubContext<SignalRHub> signalRHub)
{
_signalRHub = signalRHub;
}
protected override async Task ExecuteAsync(CancellationToken stoppingToken)
{
while (!stoppingToken.IsCancellationRequested)
{
try
{
Console.WriteLine($"{DateTime.Now}:服务已启动");
Random rnd = new Random();
int score = rnd.Next(60, 100);
string[] students = { "段誉", "萧峰", "虚竹", "阿朱", "阿紫", "王语嫣", "木婉清", "钟灵", "阿碧", "慕容复", "段正淳", "鸠摩智" };
var name = students[rnd.Next(0, students.Length)];
await _signalRHub.Clients.All.SendAsync("ReceiveScore", name, score, $"{name}的成绩是{score}");
Console.WriteLine($"{DateTime.Now}:发布成绩:{name}获取{score}分");
await Task.Delay(4000, stoppingToken);
}
catch (Exception ex)
{
Console.WriteLine($"服务异常:{ex.Message}");
}
}
}
}
}
builder.Services.AddHostedService<WorkerService>();
app.MapHub<SignalRHub>("/hubs/score");
启动项目,查看效果:
SignalR服务端搭建完毕,接下来,实现SignalR的客户端,用控制台程序实现。
同样的方法安装SignalR客户端包【Microsoft.AspNetCore.SignalR.Client】,
在Program.cs中添加代码:
using Microsoft.AspNetCore.SignalR.Client;
//here is SignalR Sender URL
string hubUrl = "http://localhost:5000/hubs/score";
var hubConnection = new HubConnectionBuilder().WithUrl(hubUrl).Build();
// Register a handler for messages from the SignalR hub
// "ReceiveStockPrice" is the topic to which SignalR sending the singnals
hubConnection.On<string, int, string>("ReceiveScore", (name, score, msg) =>
{
Console.WriteLine($"接收消息--> 学生:{name} 成绩:{score} 备注:{msg}");
});
try
{
// Start the connection
hubConnection.StartAsync().Wait();
Console.WriteLine("SignalR 已连接到服务器");
}
catch (Exception ex)
{
Console.WriteLine($"SignalR 连接服务异常:{ex.Message}");
throw;
}
CancellationTokenSource cancellationTokenSource = new CancellationTokenSource();
var cancellationToken = cancellationTokenSource.Token;
Console.CancelKeyPress += (sender, a) =>
{
a.Cancel = true;
Console.WriteLine("SignalR 停止连接");
cancellationTokenSource.Cancel();
};
try
{
await Task.Delay(Timeout.Infinite, cancellationToken);
}
catch (TaskCanceledException)
{
}
await hubConnection.StopAsync();
Console.WriteLine("SignalR 连接已关闭");
启动项目,查看效果:
本文章转载微信公众号@武穆逸仙