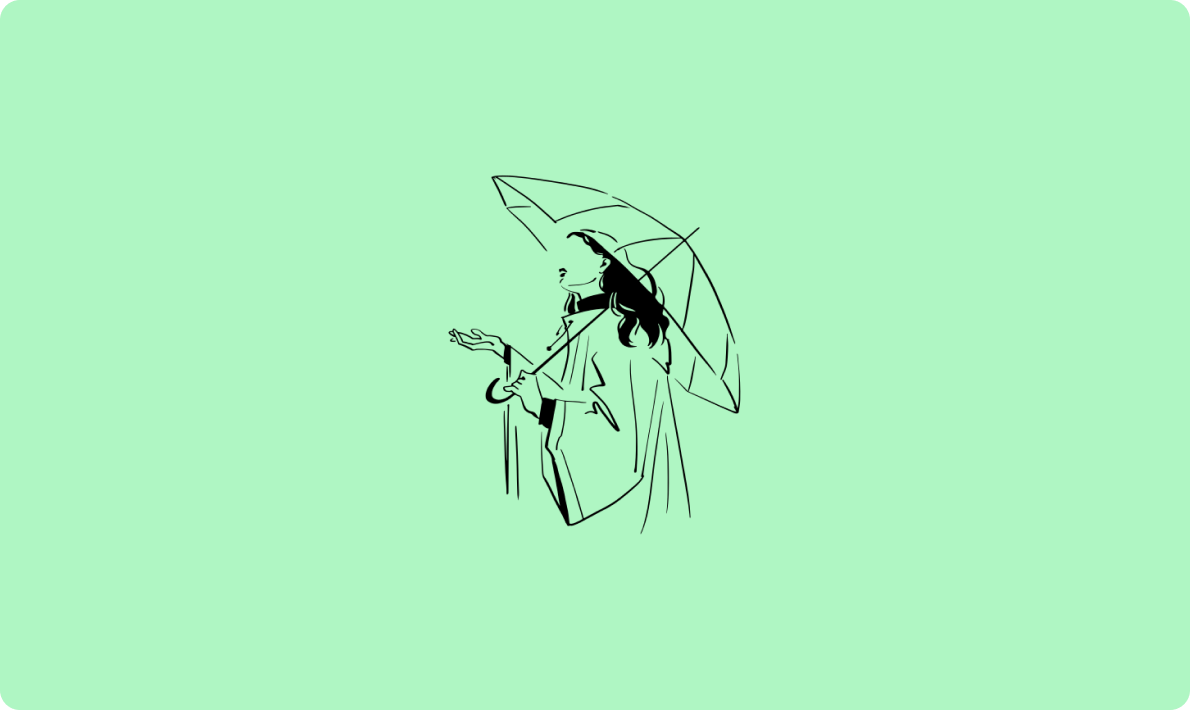
Python调用墨迹天气API实践指南
在本文中,我将以实用且客观的方式讨论Spring 框架与市场上主要的人工智能产品之一 OpenAI API 的资源的集成。
人工智能资源的使用在许多产品中变得越来越必要,因此,通过 Spring 框架在Java解决方案中展示其应用可以使大量当前正在生产的项目从该资源中受益。
该项目使用的所有代码均可通过 GitHub 获取。要下载它,只需运行以下命令:
git clone https://github.com/felipecaparelli/openai-spring.git或通过 SSL git clone。
注意:请务必注意,使用 OpenAI 帐户使用此 API 会产生费用。请确保您了解与每个请求相关的价格(它会因用于请求和在响应中显示的令牌而异)。
根据官方文档的定义,首先,您需要一个来自 OpenAI 的 API 密钥才能使用 GPT 模型。如果您没有帐户,请在 OpenAI 的网站上注册,然后从 API 仪表板创建一个 API 密钥。
准备项目结构的最简单方法是通过名为Spring Initializr的 Spring 工具。它将生成项目的基本框架,添加必要的库、配置以及启动应用程序的主类。您必须至少选择Spring Web依赖项。在项目类型中,我选择了 Maven 和Java 17。我还包含了库,httpclient5
因为需要配置我们的 SSL 连接器。
按照生成的pom.xml的片段进行操作:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>3.3.2</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>br.com.erakles</groupId>
<artifactId>spring-openai</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>spring-openai</name>
<description>Demo project to explain the Spring and OpenAI integration</description>
<properties>
<java.version>17</java.version>
<spring-ai.version>1.0.0-M1</spring-ai.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents.client5</groupId>
<artifactId>httpclient5</artifactId>
<version>5.3.1</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
在您的配置文件(application.properties)中,在属性中设置 OpenAI 密钥openai.api.key
。您还可以在属性文件中替换模型版本以使用不同的 API 版本,例如gpt-4o-mini
。
spring.application.name=spring-openai
openai.api.url=https://api.openai.com/v1/chat/completions
openai.api.key=YOUR-OPENAI-API-KEY-GOES-HERE
openai.api.model=gpt-3.5-turbo
通过 Java 连接此服务的一个棘手部分是,默认情况下,它将要求您的 HTTP 客户端在执行此请求时使用有效证书。为了解决这个问题,我们将跳过此验证步骤。
要禁用 JDK 对 HTTPS 请求所需的安全证书要求,您必须通过配置类在RestTemplate bean 中包含以下修改:
import org.apache.hc.client5.http.classic.HttpClient;
import org.apache.hc.client5.http.impl.classic.HttpClients;
import org.apache.hc.client5.http.impl.io.BasicHttpClientConnectionManager;
import org.apache.hc.client5.http.socket.ConnectionSocketFactory;
import org.apache.hc.client5.http.socket.PlainConnectionSocketFactory;
import org.apache.hc.client5.http.ssl.NoopHostnameVerifier;
import org.apache.hc.client5.http.ssl.SSLConnectionSocketFactory;
import org.apache.hc.core5.http.config.Registry;
import org.apache.hc.core5.http.config.RegistryBuilder;
import org.apache.hc.core5.ssl.SSLContexts;
import org.apache.hc.core5.ssl.TrustStrategy;
import org.springframework.boot.web.client.RestTemplateBuilder;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.http.client.HttpComponentsClientHttpRequestFactory;
import org.springframework.web.client.RestTemplate;
import javax.net.ssl.SSLContext;
@Configuration
public class SpringOpenAIConfig {
@Bean
public RestTemplate secureRestTemplate(RestTemplateBuilder builder) throws Exception {
// This configuration allows your application to skip the SSL check
final TrustStrategy acceptingTrustStrategy = (cert, authType) -> true;
final SSLContext sslContext = SSLContexts.custom()
.loadTrustMaterial(null, acceptingTrustStrategy)
.build();
final SSLConnectionSocketFactory sslsf =
new SSLConnectionSocketFactory(sslContext, NoopHostnameVerifier.INSTANCE);
final Registry<ConnectionSocketFactory> socketFactoryRegistry =
RegistryBuilder.<ConnectionSocketFactory>create()
.register("https", sslsf)
.register("http", new PlainConnectionSocketFactory())
.build();
final BasicHttpClientConnectionManager connectionManager =
new BasicHttpClientConnectionManager(socketFactoryRegistry);
HttpClient client = HttpClients.custom()
.setConnectionManager(connectionManager)
.build();
return builder
.requestFactory(() -> new HttpComponentsClientHttpRequestFactory(client))
.build();
}
}
现在我们已经准备好了所有配置,是时候实现一个处理与 ChatGPT API 通信的服务了。我正在使用 Spring 组件RestTemplate
,它允许执行对 OpenAI 端点的 HTTP 请求。
import org.springframework.beans.factory.annotation.Value;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpMethod;
import org.springframework.http.MediaType;
import org.springframework.stereotype.Service;
import org.springframework.web.client.RestTemplate;
@Service
public class JavaOpenAIService {
@Value("${openai.api.url}")
private String apiUrl;
@Value("${openai.api.key}")
private String apiKey;
@Value("${openai.api.model}")
private String modelVersion;
private final RestTemplate restTemplate;
public JavaOpenAIService(RestTemplate restTemplate) {
this.restTemplate = restTemplate;
}
/**
* Sends a request to the OpenAI API with the given prompt and returns the response.
*
* @param prompt the question to ask the AI
* @return the response in JSON format
*/
public String ask(String prompt) {
HttpEntity<String> entity = new HttpEntity<>(buildMessageBody(modelVersion, prompt), buildOpenAIHeaders());
return restTemplate
.exchange(apiUrl, HttpMethod.POST, entity, String.class)
.getBody();
}
private HttpHeaders buildOpenAIHeaders() {
HttpHeaders headers = new HttpHeaders();
headers.set("Authorization", "Bearer " + apiKey);
headers.set("Content-Type", MediaType.APPLICATION_JSON_VALUE);
return headers;
}
private String buildMessageBody(String modelVersion, String prompt) {
return String.format("{ \"model\": \"%s\", \"messages\": [{\"role\": \"user\", \"content\": \"%s\"}]}", modelVersion, prompt);
}
}
然后,您可以创建自己的 REST API 来接收问题并将其重定向到您的服务。
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import br.com.erakles.springopenai.service.SpringOpenService;
@RestController
public class SpringOpenAIController {
private final SpringOpenService springOpenService;
SpringOpenAIController(SpringOpenService springOpenService) {
this.springOpenService = springOpenService;
}
@GetMapping("/chat")
public ResponseEntity<String> sendMessage(@RequestParam String prompt) {
return ResponseEntity.ok(springOpenService.askMeAnything(prompt));
}
}
这些是将您的 Web 应用程序与 OpenAI 服务集成所需的步骤,因此您以后可以通过添加更多功能(例如向其端点发送语音、图像和其他文件)来改进它。
启动 Spring Boot 应用程序 ( ./mvnw spring-boot:run
) 后,要测试 Web 服务,您必须运行以下 URL http://localhost:8080/ask?promp={add-your-question}。
如果您所有操作正确,您将能够在响应主体中读取以下结果:
{
"id": "chatcmpl-9vSFbofMzGkLTQZeYwkseyhzbruXK",
"object": "chat.completion",
"created": 1723480319,
"model": "gpt-3.5-turbo-0125",
"choices": [
{
"index": 0,
"message": {
"role": "assistant",
"content": "Scuba stands for \"self-contained underwater breathing apparatus.\" It is a type of diving equipment that allows divers to breathe underwater while exploring the underwater world. Scuba diving involves using a tank of compressed air or other breathing gas, a regulator to control the flow of air, and various other accessories to facilitate diving, such as fins, masks, and wetsuits. Scuba diving allows divers to explore the underwater environment and observe marine life up close.",
"refusal": null
},
"logprobs": null,
"finish_reason": "stop"
}
],
"usage": {
"prompt_tokens": 12,
"completion_tokens": 90,
"total_tokens": 102
},
"system_fingerprint": null
}
希望本教程能帮助您首次与 OpenAI 互动,让您在深入 AI 之旅时生活更轻松。
原文链接:https://dzone.com/articles/integrate-spring-with-open-ai