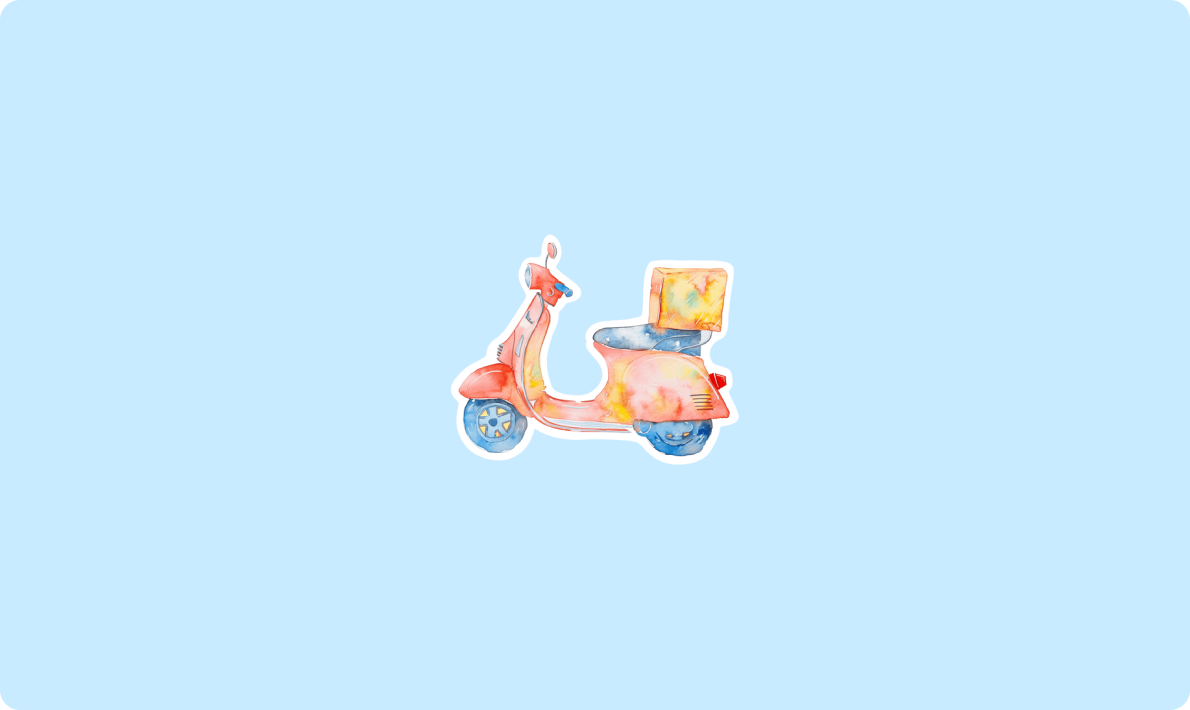
如何调用 Minimax 的 API
本文将深入探讨Firstup API接口的使用方法,涵盖API的基本概念、认证机制、常见接口的使用示例以及如何通过代码实现与Firstup平台的交互。本文假设读者具备一定的编程基础,熟悉RESTful API和常见的编程语言(如Python)。
Firstup API是一组基于RESTful架构的接口,允许开发者通过HTTP请求与Firstup平台进行交互。通过这些接口,开发者可以实现以下功能:
Firstup API的核心优势在于其灵活性和可扩展性。企业可以根据自身需求,定制化开发与Firstup平台的集成方案,从而提升员工沟通的效率和效果。
在使用Firstup API之前,首先需要进行身份认证。Firstup API采用OAuth 2.0协议进行认证和授权。OAuth 2.0是一种广泛使用的开放标准,允许第三方应用在用户授权的情况下访问受保护的资源。
要访问Firstup API,首先需要获取访问令牌(Access Token)。以下是获取访问令牌的步骤:
client_id
和client_secret
。client_id
和client_secret
向Firstup的认证服务器发送请求,获取访问令牌。以下是一个使用Python获取访问令牌的示例代码:
import requests
# Firstup认证服务器的URL
auth_url = "https://api.firstup.io/oauth/token"
# 应用的client_id和client_secret
client_id = "your_client_id"
client_secret = "your_client_secret"
# 请求参数
data = {
"grant_type": "client_credentials",
"client_id": client_id,
"client_secret": client_secret
}
# 发送POST请求获取访问令牌
response = requests.post(auth_url, data=data)
# 解析响应
if response.status_code == 200:
token_data = response.json()
access_token = token_data["access_token"]
print("Access Token:", access_token)
else:
print("Failed to get access token:", response.status_code, response.text)
获取到访问令牌后,可以在后续的API请求中使用该令牌进行身份验证。通常,访问令牌需要放在HTTP请求的Authorization
头中。
以下是一个使用访问令牌调用Firstup API的示例:
import requests
# Firstup API的基础URL
base_url = "https://api.firstup.io/v1"
# 访问令牌
access_token = "your_access_token"
# 设置请求头
headers = {
"Authorization": f"Bearer {access_token}",
"Content-Type": "application/json"
}
# 调用API获取用户信息
response = requests.get(f"{base_url}/users", headers=headers)
# 解析响应
if response.status_code == 200:
users = response.json()
print("Users:", users)
else:
print("Failed to get users:", response.status_code, response.text)
Firstup API提供了丰富的接口,涵盖了用户管理、内容管理、通知发送等多个方面。以下是一些常见API接口的使用示例。
通过/users
接口,可以获取平台上的用户列表。
import requests
# Firstup API的基础URL
base_url = "https://api.firstup.io/v1"
# 访问令牌
access_token = "your_access_token"
# 设置请求头
headers = {
"Authorization": f"Bearer {access_token}",
"Content-Type": "application/json"
}
# 调用API获取用户列表
response = requests.get(f"{base_url}/users", headers=headers)
# 解析响应
if response.status_code == 200:
users = response.json()
print("Users:", users)
else:
print("Failed to get users:", response.status_code, response.text)
通过/users/{user_id}
接口,可以获取指定用户的详细信息。
import requests
# Firstup API的基础URL
base_url = "https://api.firstup.io/v1"
# 访问令牌
access_token = "your_access_token"
# 用户ID
user_id = "12345"
# 设置请求头
headers = {
"Authorization": f"Bearer {access_token}",
"Content-Type": "application/json"
}
# 调用API获取用户信息
response = requests.get(f"{base_url}/users/{user_id}", headers=headers)
# 解析响应
if response.status_code == 200:
user = response.json()
print("User:", user)
else:
print("Failed to get user:", response.status_code, response.text)
通过/contents
接口,可以获取平台上的内容列表。
import requests
# Firstup API的基础URL
base_url = "https://api.firstup.io/v1"
# 访问令牌
access_token = "your_access_token"
# 设置请求头
headers = {
"Authorization": f"Bearer {access_token}",
"Content-Type": "application/json"
}
# 调用API获取内容列表
response = requests.get(f"{base_url}/contents", headers=headers)
# 解析响应
if response.status_code == 200:
contents = response.json()
print("Contents:", contents)
else:
print("Failed to get contents:", response.status_code, response.text)
通过/contents
接口,可以创建新的内容。
import requests
# Firstup API的基础URL
base_url = "https://api.firstup.io/v1"
# 访问令牌
access_token = "your_access_token"
# 设置请求头
headers = {
"Authorization": f"Bearer {access_token}",
"Content-Type": "application/json"
}
# 新内容的数据
new_content = {
"title": "New Content Title",
"body": "This is the body of the new content.",
"channels": ["channel_id_1", "channel_id_2"]
}
# 调用API创建新内容
response = requests.post(f"{base_url}/contents", headers=headers, json=new_content)
# 解析响应
if response.status_code == 201:
created_content = response.json()
print("Created Content:", created_content)
else:
print("Failed to create content:", response.status_code, response.text)
通过/notifications
接口,可以向指定用户或用户组发送通知。
import requests
# Firstup API的基础URL
base_url = "https://api.firstup.io/v1"
# 访问令牌
access_token = "your_access_token"
# 设置请求头
headers = {
"Authorization": f"Bearer {access_token}",
"Content-Type": "application/json"
}
# 通知数据
notification_data = {
"title": "Important Notification",
"body": "This is an important notification for all employees.",
"recipients": ["user_id_1", "user_id_2"],
"channels": ["channel_id_1"]
}
# 调用API发送通知
response = requests.post(f"{base_url}/notifications", headers=headers, json=notification_data)
# 解析响应
if response.status_code == 201:
notification = response.json()
print("Notification Sent:", notification)
else:
print("Failed to send notification:", response.status_code, response.text)
在使用Firstup API时,可能会遇到各种错误。常见的错误包括:
为了确保API调用的稳定性,建议在代码中加入错误处理机制。以下是一个简单的错误处理示例:
import requests
# Firstup API的基础URL
base_url = "https://api.firstup.io/v1"
# 访问令牌
access_token = "your_access_token"
# 设置请求头
headers = {
"Authorization": f"Bearer {access_token}",
"Content-Type": "application/json"
}
try:
# 调用API获取用户列表
response = requests.get(f"{base_url}/users", headers=headers)
response.raise_for_status() # 抛出HTTP错误
users = response.json()
print("Users:", users)
except requests.exceptions.HTTPError as err:
print(f"HTTP error occurred: {err}")
except Exception as err:
print(f"Other error occurred: {err}")
Firstup API为企业提供了强大的通信工具,通过灵活的接口和丰富的功能,开发者可以轻松地将Firstup平台集成到现有的企业系统中。本文详细介绍了Firstup API的基本概念、认证机制、常见接口的使用方法以及错误处理技巧,并提供了Python代码示例,帮助开发者快速上手。
通过合理利用Firstup API,企业可以实现自动化、定制化的通信解决方案,提升员工沟通的效率和效果,从而推动企业的数字化转型。